WKT for what? A deeper dive into Well-Known Text format for API calls & testing

Lately, I’ve frequently found myself creating a bounding box for an area of interest when sending API requests. This mainly has been when using our Traffic Analytics API which, as the name implies, is a traffic data REST service. It has a ‘queryFilter’ to set specific conditions, of which is a location parameter where the geometry can be defined. There I can specify a string in WKT format describing a polygon or multipolygon for a geospatial query:
{
"queryFilter": {
"location": {
"geometry": " POLYGON ((-120.95430135726929 43.96672178337354,-121.00457936525343 37.6918288036601,-116.05131715536116 36.96501709299666,-115.04165858030319 44.12169665426504,-120.95430135726929 43.96672178337354))
},
This string/text markup is just a small bounding box that represents vector-based geometric objects and looks like this:

It got me thinking too much about WKT. Where did this format originate? I then remembered an older blog post that is nearing its 4 year anniversary, which, for the longest time, seemed to perform really well in terms of page views/unique visitors. The success of that blog perhaps came down to the simplicity of describing a multifaceted topic easily and then instructing you on how to create them for your own HERE API projects.
I thought it would be interesting to go a little deeper on the subject to answer some of my questions all while highlighting recent use cases. Consider this part research, part resource guide and part Q/A. For example:
- What is the format’s origin story?
- How many types (geometries) are there and why/when would I use it?
- Why it matters in the context of HERE Location Services.
- How many ways can one construct/make a WKT (string or file)?
- Best ways to validate its formatted correctly?
- What limitations does it have?
Well-Known What?
Let’s backup and talk about what WKT is and where it came from. Beyond an acronym, it is simply a text representation (markup language) of geographic features. This can be point, polygon, multipolygon and others (it would appear in total 18 different Geometry types with 4 variations on each (2D, Z, M, ZM). Though our primary interest is in polygon/multipolygon for geospatial queries (API calls).
Not surprisingly, back in 1999 WKT (as well as WKT-CRS) was defined by the Open Geospatial Consortium (OGC). The definition has changed and is more involved but you can be read about here. WKT is well-established in GIS to describe the geometry of geographic features as vector data, which also includes web app/map development and APIs. WKT is interchangeable so in theory it makes data exchange easy among various software.
As an example, a polygon and multipolygon should in theory be 4 vertices (plural of vertex). Each vertex or “node” is a just a coordinate pair ((x1 y1, x2 y2, …)) and even a triangle needs a 4th pair to be “closed." In other words, each pair of x and y values represents a single vertex of the polygon. The general rule is that it needs to be topologically closed to be a polygon:
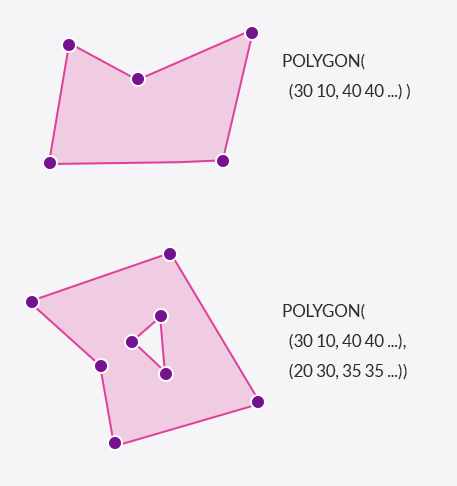
At HERE, we basically are looking at WKT as a subclass of a Geometry class which can be used to visualize WKT data on a Map.
So why would I use/need one?
There are a few ways to think about this. In the context of geospatial queries within RESTful API calls, using WKT as a string format to represent geometric objects offers some advantages:
- It's standardized = facilitates interoperability. WKT is a good choice for data exchange between different systems or applications in a RESTful API context.
- Readability: WKT's syntax is relatively easy to understand and write. A polygon can be easily described, making it more accessible for developers to work with compared to binary or less intuitive formats.
- When used in RESTful API calls, transmitting spatial data as WKT strings can be more efficient than using more verbose formats. While binary formats like Well-Known Binary (WKB) can offer size advantages for more complex geometries, WKT strikes a balance between readability and efficiency for many use cases.
- Ease of Use with SQL and Databases: Many spatial databases (such as PostGIS for PostgreSQL) support WKT directly.
- Compatibility with Web Standards: WKT can be easily incorporated into the body of POST requests or, for simpler geometries, appended to GET request URLs (less common). See more on this below.
With HERE specifically
In a HERE web app development/testing and when making requests via tools like Postman context, using WKT is easy. These are a few use cases:
- API Request Testing: When using Postman to test HERE APIs, WKT strings can be included in the body of POST requests to test APIs that accept geospatial data:
{
"geometry": "POLYGON((10 10, 20 20, 30 10, 40 40, 50 50, 60 60, 10 10))"
}
- Simplified Geospatial Data Representation- HERE's Geofencing API can use WKT for defining the shapes of geofenced areas. When setting up a geofence in a web application, you can specify the area using WKT, making it straightforward to define complex polygons that represent restricted or monitored zones:
ID NAME ABBR WKT
1 Alexanderplatz AL POLYGON((13.41252 52.52228,13.41426 52.5221,13.41522,52.52113,13.41227,52.51981))
- For applications that require custom routing or waypoint optimization, WKT can represent start, end points, or waypoints. Although HERE APIs typically use latitude and longitude pairs for routing requests, WKT can be useful in pre-processing or post-processing steps, especially when dealing with complex geometric operations or when integrating with other systems that use WKT.
- WKT can be used to define the geometries of custom map features or location data, which can then be visualized on a map within your web application. Same is true with user-Generated Geospatial Content. While the HERE Maps API doesn’t directly support WKT, in web apps that allow users to define areas of interest (such as search areas or zones for property listings), WKT can be a format for users to specify these geometries, which are then used in API requests. Here is a simple web app/map I made with the HERE Maps API for JS to find WKT for any area:
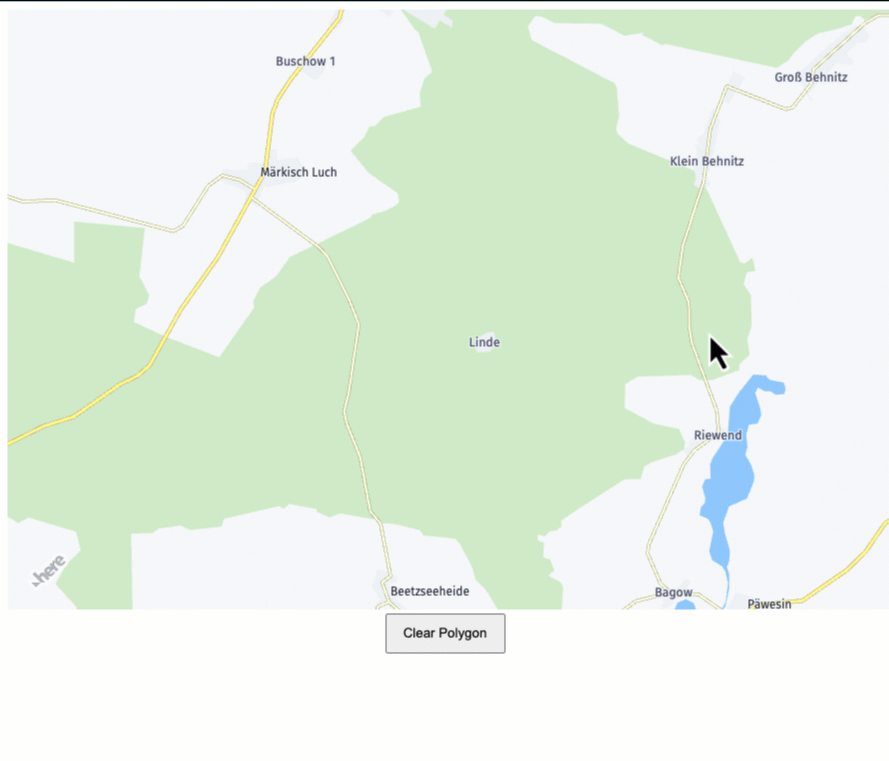
How do you make a WKT string?
The original blog outlines a super simple way to do this, and is often the case, there are many ways of making a WKT string or file. You can create a new file and use .wkt extension manually, copy the string and move on, or do it in command line but I have also included the below non-exhaustive list.
As a geo-developer these are more of the tools I would recommend:
- https://clydedacruz.github.io/openstreetmap-wkt-playground/ (validator and WKT creator)
- https://arthur-e.github.io/Wicket/sandbox-gmaps3.html
- https://www.here.com/learn/blog/create-a-wkt-file-using-here-data-layers-and-geopandas
- Using the GeoPandas library, like if you want to parse other geospatial formats
If you are a GIS practitioner, you likely would do one or more of the following:
- Use any text editor/command line.
- Within traditional GIS software like ArcGIS & QGIS during export of a layer.
- If you live in or are familiar with PostgreSQL databases, use PostGIS.
In our workflows, we often will need to convert GeoJSON, often from GIS workflows to WKT. You can then use a validation tool use methods in next section. Once you have your string you can use it for any calls/testing.
How can I validate WKT is properly formatted?
A couple of ways to validate that WKT data is structured properly:
- Use geospatial libraries such as Shapely (in Python):
pip install shapely
from shapely.wkt import loads
def is_valid_wkt(wkt_data):
try:
# Attempt to parse the WKT data
shape = loads(wkt_data)
return True
except Exception as e:
# If an error occurs, the WKT is invalid
print(f"Invalid WKT: {e}")
return False
# Example usage
wkt_data = "POLYGON ((30 10, 40 40, 20 40, 10 20, 30 10))"
print(is_valid_wkt(wkt_data)) # True for valid WKT, False for invalid
This script tries to parse the WKT string using Shapely and if it fails an exception is caught returning ‘False’, meaning the WKT is invalid. Others ways to consider:
- Terraformer JS library – is a JavaScript library that includes utilities for converting between different geographic coordinate systems and formats, including WKT.
- Online WKT Validators- Probably the easiest option as there are several. A few noteworthy ones:
Limitations?
While there are limitations compared to other geospatial formats, for our primary use case of defining a bounding box for a geospatial query it is ideal and simple. If the polygon/multi was extremely complex with many vertices (complex geometry), I could see a potential issue due to size. You also wouldn’t want to use it for web app development in most (all?) cases as it is too verbose and lacks non-spatial attributes. GeoJSON and TopoJSON are far better options.
Closing the loop
Is that everything you wanted to know about WKT? Hopefully it covers enough to demystify WKT if you weren't able to catch the previous blog back in 2020. Feel to reach out with any questions or comments on Slack! @heredev
Have your say
Sign up for our newsletter
Why sign up:
- Latest offers and discounts
- Tailored content delivered weekly
- Exclusive events
- One click to unsubscribe